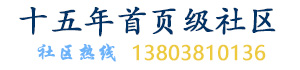
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。”需要全文内容也请联系孙老师。
An Alpha effect is something that you definitely have seen, though may be unknowingly. It's a simple but effective visual effect where images or text fade in or out to a solid background or an image. You probably have come across it if you have used Macromedia® Flash®. It's an effect that has been used extensively in movies, television programs, games and almost any visual media that exists. It's so common that it goes unnoticed.
An alpha effect is usually employed as an animation. And animations require discrete frames to be displayed in quick succession with progressive alterations in sequence. Keeping this in mind, the first part of this article explains how to create a single alpha image out of image objects. Next, it explains how you could create this effect when calling drawing methods like that of lines, arcs, text etc. The article then goes on to explain how to create an animation out of all that. Finally, it points out some little details worth to be noted.
虽然可能在不知不觉中出现了Alpha效应,但你肯定已经看过了。这是一种简单但有效的视觉效果,其中图像或文本淡入或淡出转换到纯色背景或图像。如果您使用过Macromedia®Flash®,可能会遇到它。这种效果已广泛用于电影、电视节目、游戏以及几乎所有存在的视觉媒体中。它是如此常见,以至于它被忽视了。
alpha效果通常用作动画。动画要求离散帧快速连续显示,并按顺序逐步改变。牢记这一点,本文的第一部分将介绍如何从图像对象中创建单个alpha图像。接下来,它解释了在调用绘图方法(如线,弧,文本等)时如何创建此效果。然后文章继续解释如何从中创建动画。最后,它指出了一些值得注意的细节。
The code uses the following additional namespaces:
该代码使用以下附加名称空间:
If you have been working in C# for some time, you might want to skip the subsection 'The first steps' and proceed to 'Step 1'. 'The first steps' gives you a small introduction to graphics programming.
To work with graphics or with formatted text in .NET, you usually rely on GDI+. GDI+ is simply an API (Application Programming Interface) that helps programmers work with display devices and graphics better.
To draw some graphics, you need an area where you want to work upon. Or in better words, a surface where the graphics will be drawn. These surfaces can be portions of display devices or of images themselves (this is how you modify an image programmatically). To represent a surface and to work on it, you use a Graphics object (System.Drawing namespace).
So, the first step in applying alpha is to create a Graphics object of the surface you want to draw onto.
如果您已经在C#中工作了一段时间,您可能想跳过“第一步”小节并继续执行“步骤1”。“第一步”为您提供了一些图形编程的简介。
要在.NET中使用图形或格式化文本,通常需要依赖GDI +。GDI +只是一个API(应用程序编程接口),可以帮助程序员更好地处理显示设备和图形。
要绘制一些图形,您需要一个您想要处理的区域。或者用更好的词来表示绘制图形的表面。这些表面可以是显示设备的一部分或图像本身(这是您以编程方式修改图像的方式)。要表示曲面并对其进行处理,请使用Graphics对象(System.Drawing命名空间)。
因此,应用alpha的第一步是创建Graphics要绘制的曲面的对象。
If the surface you want to work upon is a portion of a control, you call the control's CreateGraphics method to create it's Graphics object. For example, suppose you have a PictureBox control, pictureBox1 in your form; the following code will let you create it's Graphics object:
如果要处理的曲面是控件的一部分,则调用控件的CreateGraphics方法来创建它的Graphics对象。例如,假设您的表单中有一个PictureBox控件pictureBox1; 以下代码将允许您创建它的Graphics对象:
Graphics gra = pictureBox1.CreateGraphics();
If the surface you want to work upon is a portion of an image, you call the static Graphics.FromImage method to create the image object's Graphics object. Image objects are represented by instances of either the Bitmapor the Metafile class, both of which derive from the abstract Image class. For example, the following code creates a Bitmap object from a file logo.jpg, and then creates it's Graphics object.
如果要处理的曲面是图像的一部分,则调用静态Graphics.FromImage方法来创建图像对象的Graphics对象。图像对象由Bitmap或Metafile类的实例表示,这两个实例都派生自抽象Image类。例如,以下代码Bitmap从文件logo.jpg创建一个对象,然后创建它的Graphics对象。
Bitmap logo = new Bitmap("logo.jpg"); Graphics gra = Graphics.FromImage(logo);
The Graphics class contains several methods you can use to create basic graphics like drawing rectangles, filling regions and so on. But to apply alpha on images, you need more control. For this, you will use two classes: ImageAttributes and ColorMatrix (System.Drawing.Imaging namespace). An ImageAttributes object lets you control the way graphics is rendered by letting you specify different settings like color adjustment, grayscale adjustment and more. The ColorMatrix class can be considered as a helper class whose instances are parameters in most of the methods of the ImageAttributes class. It contains values specifying the Alpha, Red, Green and Blue channels.
So, the next step is to initialize a color matrix object and pass it to the appropriate method of an ImageAttributes object.
本Graphics类包含了几种方法,你可以用它来创建基本的图形像绘制矩形、填充区等。但是要在图像上应用alpha,您需要更多控制。为此,您将使用两个类:ImageAttributes和ColorMatrix(System.Drawing.Imaging命名空间)。ImageAttributes通过允许您指定不同的设置(如颜色调整,灰度调整等),可以使用对象控制图形的渲染方式。该ColorMatrix类可被视为一个辅助类,其实例出现在ImageAttributes类大多数的方法参数上。它包含指定Alpha、Red、Green和Blue通道的值。
因此,下一步是初始化颜色矩阵对象并将其传递给ImageAttributes对象的适当方法。
A few basics: An image is composed of colors. All the colors of an image is usually made up of three primary colors: Red, Green and Blue (RGB in short). Different combinations of these three primary colors create different colors which are known as composite colors. Normally, each primary color can take any value in the range of 0 to 255. When all the primary colors have the value 0, then it creates the color black. When all of them are 255, it's white. A Channel is an entity present in images, which can be thought of as what defines the visibility of a specific primary color. For example, if you cut off the Red channel, no Red color (of range 0-255) will be displayed. Additionally, an Alpha channel exists in some images, which defines the total visibility of the image. Hence, an image may have RGBA as it's channels. The primary colors, the range of values a primary color can take, the channels, etc. are all dependent on the image format used. That is off-track.
A color matrix is a matrix that contains values for channels. It's a 5x5 matrix which represents values for the Red, Green, Blue, Alpha channels and another element w, in that order (RGBAw).
In a ColorMatrix object, the diagonal elements of the matrix define the channel values viz. (0,0), (1,1), (2,2), (3,3), and (4,4), in the order as specified before - RGBAw. The values are of type float, and range from 0 to 1. The element w (at (4,4) ) is always 1.
What you have to do is to create a new ColorMatrix object and set the channel values as required. To do this, you access the properties representing the respective matrix elements. For example, the Matrix00 property lets you access the element at (0,0).
Once you initialize the ColorMatrix object, you create a new ImageAttributes object, and assign the newly created ColorMatrix object to it. This is done by calling the SetColorMatrix method on the ImageAttributes object. For example, the following code creates a ColorMatrix object, sets it's alpha value to 0.5, and creates a new ImageAttributes object, setting it's color matrix to the one just created:
一些基础知识:图像由颜色组成。图像的所有颜色通常由三种基色组成:红色,绿色和蓝色(简称RGB)。这三种基色的不同组合产生不同的颜色,称为复合颜色。通常,每种原色可以采用0到255范围内的任何值。当所有原色的值都为0时,它会产生黑色。当它们都是255时,它是白色的。通道是图像中存在的实体,可以将其视为定义特定原色的可见性的实体。例如,如果切断红色通道,则不会显示红色(范围0-255)。此外,某些图像中存在Alpha通道,它定义了图像的总体可见性。因此,图像可以具有RGBA作为其通道。原色,主要颜色可以采用的值范围,通道等都取决于所使用的图像格式。那是偏离轨道的。
颜色矩阵是包含通道值的矩阵。它是一个5x5矩阵,表示红色,绿色,蓝色,Alpha通道和另一个元素w的值,按此顺序(RGBAw)。
在一个ColorMatrix对象中,矩阵的对角元素定义了通道值即。(0,0),(1,1),(2,2),(3,3)和(4,4),按照前面指定的顺序 - RGBAw。值的类型为float,范围为0到1.元素w(at(4,4))始终为1。
您需要做的是创建一个新ColorMatrix对象并根据需要设置通道值。为此,您可以访问表示相应矩阵元素的属性。例如,该Matrix00属性允许您访问(0,0)处的元素。
初始化ColorMatrix对象后,您将创建一个新ImageAttributes对象,并将新创建的ColorMatrix对象分配给它。这是通过调用对象上的方法来完成的。例如,以下代码创建一个对象,将其alpha值设置为0.5,并创建一个新对象,将其颜色矩阵设置为刚刚创建的颜色矩阵:SetColorMatrixImageAttributesColorMatrixImageAttributes
ColorMatrix cm = new ColorMatrix(); cm.Matrix33 = 0.5; ImageAttributes ia = new ImageAttributes(); ia.SetColorMatrix(cm);
The final step is to draw the original image with the ImageAttributes object just created. Using this ImageAttributes object would draw the original image with the alpha value we set in the color matrix, creating the alpha image.最后一步是使用刚刚创建的ImageAttributes对象绘制原始图像。使用此ImageAttributes对象将使用我们在颜色矩阵中设置的alpha值绘制原始图像,从而创建alpha图像。
To draw the alpha image, we call one of the overloads of the Graphics.DrawImage method on the Graphicsobject. Note that this overload should accept an ImageAttributes object with which we would specify the render modifications. So select an appropriate overload and call it. For example, the following code draws the image ImageBitmap at the location specified, with the ImageAttributes object ia:要绘制alpha图像,我们在对象上调用方法的一个重载。请注意,此重载应接受一个对象,我们将使用该对象指定渲染修改。因此,选择适当的重载并调用它。例如,以下代码使用对象在指定的位置绘制图像:Graphics.DrawImageGraphicsImageAttributesImageBitmapImageAttributesia
gra.DrawImage(ImageBitmap, new Rectangle(0,0,ImageBitmap.Width,ImageBitmap.Height), 0,0,ImageBitmap.Width, ImageBitmap.Height, GraphicsUnit.Pixel, ia);
The following is a complete code listing for the whole set of steps we went through:以下是我们完成的整个步骤的完整代码清单:
// Get a picture box's Graphics object Graphics gra = pictureBox1.Graphics; // Create a new color matrix and set the alpha value to 0.5 ColorMatrix cm = new ColorMatrix(); cm.Matrix00 = cm.Matrix11 = cm.Matrix22 = cm.Matrix44 = 1; cm.Matrix33 = 0.5; // Create a new image attribute object and set the color matrix to // the one just created ImageAttributes ia = new ImageAttributes(); ia.SetColorMatrix(cm); // Draw the original image with the image attributes specified gra.DrawImage(ImageBitmap, new Rectangle(0,0,ImageBitmap.Width, ImageBitmap.Height), 0,0,ImageBitmap.Width, ImageBitmap.Height, GraphicsUnit.Pixel, ia);
The Graphics class consists of several specific draw methods like that of lines, arcs etc. When you use such methods, it is possible to apply alpha right when you use them, rather than applying it to the image in total as explained above. This would undoubtedly be more straightforward illustrated as follows:
All the specific graphic construct draw methods of the Graphics class (except DrawIcon and DrawImage) makes use of either a Pen or a Brush object as an argument. Both the Pen and Brush classes have a common underlying element - Color; which means that both these classes use a Color structure either directly or indirectly. We make use of this fact to make the application of alpha simpler.
A Color structure defines not only the RGB channels, but also the Alpha channel. To create a new Color object together defining it's alpha, we use the Color.FromArgb method:
该Graphics类由若干具体绘制方法组成,比如线,弧等。当你使用这种方法,有可能你使用的时候,有可能使用alpha方法。毫无疑问,如下这将更直接地说明:
类的所有特定图形构造绘制方法Graphics(除了DrawIcon和DrawImage)都使用Pen或Brush对象作为参数。两个Pen和Brush类都具有共同的基本元件- Color; 这意味着这两个类Color直接或间接使用结构。我们利用这个事实使alpha的应用更简单。
甲Color结构定义不仅RGB通道,而且还Alpha通道。要创建一个新Color对象,一起定义它的alpha,我们使用以下Color.FromArgb方法:
public static Color FromArgb( int alpha, int red, int green, int blue );
where the value range of each element is 0-255.
The idea here is, to initialize a Color object with the desired alpha value, to create the appropriate Pen or Brushobjects from this Color structure, and to call the specific draw method passing the Pen or Brush object just created. This would draw the graphic with the alpha value set in the Color structure, and would create the same alpha effect as you would have obtained using the first procedure.
To create a Pen object on the basis of a Color object, use the Pen.Pen(Color) constructor.
To create a Brush object on the basis of a Color object, use the SolidBrush.SolidBrush(Color)constructor. The SolidBrush class is derived from the abstract Brush class. There are other classes derived from the Brush class some of which use the Color structure; but that's a different story.
The following code draws a string 'Text' in black with alpha 100. (Note that 100 is not 100% in this procedure. This is likely to go unnoticed.)
其中每个元素的值范围是0-255。
这里的想法是,初始化一个Color对象具有期望的α值,以创建相应的Pen或Brush从该物体Color结构,并调用特定draw方法传递Pen或Brush刚创建的对象。这将绘制具有Color结构中设置的alpha值的图形,并将创建与使用第一个过程获得的相同的alpha效果。
要基于Pen对象创建Color对象,请使用Pen.Pen(Color)构造函数。
要基于Brush对象创建Color对象,请使用SolidBrush.SolidBrush(Color)构造函数。本SolidBrush类是从抽象的派生Brush类。从类派生的其他类中有Brush一些使用该Color结构; 但这是一个不同的故事。
以下代码使用alpha 100绘制黑色字符串'Text'。(请注意,此过程中100不是100%。这可能会被忽视。)
gra = e.Graphics; gra.DrawString("Text", new Font("Verdana", 24), new SolidBrush(Color.FromArgb(100,0,0,0)), 0,0 );
In a similar manner, alpha effect on other constructs can be carried out.以类似的方式,可以进行对其他构建体的α效应。
An animation exhibiting alpha effect is usually of two types: fade-in and fade-out. A fade-in animation starts with a black (usually) background and ends with the actual image. Technically, the starting frame is of alpha zero, and the ending frame is of alpha 100. A fade-out animation is the exact opposite. The background may also be another image.
To create an animation of either of the two types, you need to create a whole sequence of such alpha images and display them in order.
First, decide on the number of discrete alpha images you need. To create a smooth effect, a minimum of fifteen frames is recommended. Once you have decided on the number, create a Bitmap array with this size. Then start a loop, and create Bitmap objects of the required alpha value within the array. Finally, depending on the destination, either create a Graphics object and draw the image, or set the control's Image property to this object. This method of creating an image sequence in the memory and then displaying them is known as Double Buffering.
The following code creates an image sequence in the procedure as mentioned above:
呈现alpha效果的动画通常有两种类型:淡入和淡出。淡入动画以黑色(通常)背景开始,以实际图像结束。从技术上讲,起始帧是alpha零,结束帧是alpha 100.淡出动画恰恰相反。背景也可以是另一图像。
要创建两种类型之一的动画,您需要创建一系列此类alpha图像并按顺序显示它们。
首先,确定所需的离散alpha图像的数量。要创建平滑效果,建议至少使用十五帧。确定数字后,创建一个具有此大小的Bitmap数组。然后启动循环,并Bitmap在数组中创建所需alpha值的对象。最后,根据目标,创建Graphics对象并绘制图像,或将控件的Image属性设置为此对象。这种在存储器中创建图像序列然后显示它们的方法称为双缓冲。
以下代码在上面提到的过程中创建一个图像序列:
int FrameCount = 15; float opacity = 0.0F; Bitmap original = new Bitmap("logo.jpg"); Bitmap[] frames = new Bitmap[FrameCount]; for(int x=0; x<FrameCount; x++, opacity+=0.1F) { Bitmap alpha = new Bitmap(original, original.Size); Graphics gra = Graphics.FromImage(alpha); gra.Clear(Color.Transparent); ColorMatrix cm = new ColorMatrix(); cm.Matrix00 = cm.Matrix11 = cm.Matrix22 = cm.Matrix44 = 1; cm.Matrix33 = opacity; ImageAttributes ia = new ImageAttributes(); ia.SetColorMatrix(cm); gra.DrawImage(original, new Rectangle(0,0,original.Width,original.Height), 0,0,original.Width, original.Height, GraphicsUnit.Pixel, ia); frames[x] = alpha; }
For displaying them, you might want to use a timer, or the control's unique paint handler.要显示它们,您可能需要使用计时器或控件的唯一绘制处理程序。