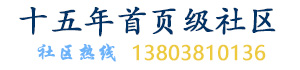
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。需要全文内容也请联系孙老师。
In this article, I'll give a demo of how to crop an image by selecting a region with the mouse.在本文中,我将演示如何通过使用鼠标选择区域来裁剪图像。
We select a region of an image, create another image of the selected region, and zoom the new image to the size of the PictureBox.
Although the PictureBox has its the SizeMode = PictureBoxSizeMode.Zoom, a MemoryOutOfRangeexception may be thrown when cropping the image several times. So, I assume this property is just for fitting an image to a PictureBox once when loading the form.我们选择图像的一个区域,创建所选区域的另一个图像,然后将新图像缩放到PictureBox大小。
虽然PictureBox有它的SizeMode = PictureBoxSizeMode.Zoom,但是在多次MemoryOutOfRange裁剪图像时可能会抛出异常。因此,我假设此属性仅用于PictureBox在加载窗体时将图像拟合到一次。
I've implemented the cropping and fitting to the PictureBox as an extension to the Image class.我已经实现了裁剪和拟合PictureBox作为Image类的扩展。
The image is cropped by cloning a region of the original image.通过克隆原始图像的区域来裁剪图像。
/// <summary>
/// Crops an image according to a selection rectangel
/// </summary>
/// <param name="image">
/// the image to be cropped
/// </param>
/// <param name="selection">
/// the selection
/// </param>
/// <returns>
/// cropped image
/// </returns>
public static Image Crop(this Image image, Rectangle selection)
{
Bitmap bmp = image as Bitmap;
// Check if it is a bitmap:
if (bmp == null)
throw new ArgumentException("No valid bitmap");
// Crop the image:
Bitmap cropBmp = bmp.Clone(selection, bmp.PixelFormat);
// Release the resources:
image.Dispose();
return cropBmp;
}
As mentioned under the Background section, fitting can't be done by the PictureBox itself. So, I've coded this myself.
As the first step, the scale factors in the vertical and horizontal directions are calculated. To scale (or zoom) the image so that the original ratio of width to height is maintained, the bigger ratio of the scale is used. The interpolation-mode is set to produce high quality pictures.如背景部分所述,拟合不能由其PictureBox自身完成。所以,我自己编写了这个。
作为第一步,计算垂直和水平方向上的比例因子。为了缩放(或缩放)图像以保持宽度与高度的原始比率,使用较大的比例比。插值模式设置为产生高质量图像。
/// <summary>
/// Fits an image to the size of a picturebox
/// </summary>
/// <param name="image">
/// image to be fit
/// </param>
/// <param name="picBox">
/// picturebox in that the image should fit
/// </param>
/// <returns>
/// fitted image
/// </returns>
/// <remarks>
/// Although the picturebox has the SizeMode-property that offers
/// the same functionality an OutOfMemory-Exception is thrown
/// when assigning images to a picturebox several times.
///
/// AFAIK the SizeMode is designed for assigning an image to
/// picturebox only once.
/// </remarks>
public static Image Fit2PictureBox(this Image image, PictureBox picBox)
{
Bitmap bmp = null;
Graphics g;
// Scale:
double scaleY = (double)image.Width / picBox.Width;
double scaleX = (double)image.Height / picBox.Height;
double scale = scaleY < scaleX ? scaleX : scaleY;
// Create new bitmap:
bmp = new Bitmap(
(int)((double)image.Width / scale),
(int)((double)image.Height / scale));
// Set resolution of the new image:
bmp.SetResolution(
image.HorizontalResolution,
image.VerticalResolution);
// Create graphics:
g = Graphics.FromImage(bmp);
// Set interpolation mode:
g.InterpolationMode = InterpolationMode.HighQualityBicubic;
// Draw the new image:
g.DrawImage(
image,
new Rectangle( // Destination
0, 0,
bmp.Width, bmp.Height),
new Rectangle( // Source
0, 0,
image.Width, image.Height),
GraphicsUnit.Pixel);
// Release the resources of the graphics:
g.Dispose();
// Release the resources of the origin image:
image.Dispose();
return bmp;
}
To restore the original image, it is saved in the form-load event. Notice, save a copy of the image and not a reference. In the latter case, the copy would point to the (cropped) image in the PictureBox. Also, on restoring, a copy is assigned to the PictureBox.要恢复原始图像,它将保存在窗体加载事件中。请注意,保存图像的副本而不是参考。在后一种情况下,副本将指向PictureBox内的(裁剪)图像。此外,在还原时,会将副本分配给PictureBox。
private Image _originalImage;
private void Form1_Load(object sender, System.EventArgs e)
{
// Save just a copy of the image on no reference!
_originalImage = pictureBox1.Image.Clone() as Image;
}
private void button1_Click(object sender, System.EventArgs e)
{
pictureBox1.Image = _originalImage.Clone() as Image;
}
Selecting a region is straightforward. Just use the MouseDown and MouseMove events.选择一个区域很简单。只需使用MouseDown和MouseMove事件。
private bool _selecting;
private Rectangle _selection;
private void pictureBox1_MouseDown(object sender, MouseEventArgs e)
{
// Starting point of the selection:
if (e.Button == MouseButtons.Left)
{
_selecting = true;
_selection = new Rectangle(new Point(e.X, e.Y), new Size());
}
}
private void pictureBox1_MouseMove(object sender, MouseEventArgs e)
{
// Update the actual size of the selection:
if (_selecting)
{
_selection.Width = e.X - _selection.X;
_selection.Height = e.Y - _selection.Y;
// Redraw the picturebox:
pictureBox1.Refresh();
}
}
To display the selection, a rectangle is drawn on the picture.要显示选择,将在图片上绘制一个矩形。
private void pictureBox1_Paint(object sender, PaintEventArgs e) { if (_selecting) { // Draw a rectangle displaying the current selection Pen pen = Pens.GreenYellow; e.Graphics.DrawRectangle(pen, _selection); } }
The end of selection is indicated by a MouseUp. Before cropping, the size of the selection is validated because it could be zero in the case of double-clicking in the PictureBox.MouseUp时选择结束。在裁剪之前,选择的大小是有效的,因为在双击的情况下它可能为零。
private void pictureBox1_MouseUp(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left &&
_selecting &&
_selection.Size != new Size())
{
// Create cropped image:
Image img = pictureBox1.Image.Crop(_selection);
// Fit image to the picturebox:
pictureBox1.Image = img.Fit2PictureBox(pictureBox1);
_selecting = false;
}
else
_selecting = false;
}