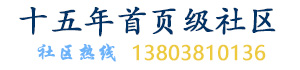
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。需要全文内容也请联系孙老师。
I found a way around the limitation :P 我找到一个解决周围限制的方法:P
I made a solution to measuring more then 32 characters
我做了一个解决方案,以测量超过32个字符
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; using System.Runtime.InteropServices; namespace DrawStringOneCharAtATime { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private Color RandomColor() { Random random = new Random(); return Color.FromArgb(random.Next(256), random.Next(256), random.Next(256)); } private Color InvertColor(Color color) { byte bRed = (byte)~(color.R); byte bGreen = (byte)~(color.G); byte bBlue = (byte)~(color.B); return Color.FromArgb(bRed, bGreen, bBlue); //return Color.FromArgb(255 - color.R, 255 - color.G, 255 - color.B); } protected override void OnPaint(PaintEventArgs e) { base.OnPaint(e); if (this.DesignMode) return; using (SolidBrush foreColorSolidBrush = new SolidBrush(this.ForeColor)) using (SolidBrush backColorSolidBrush = new SolidBrush(Color.Yellow)) { string text = "Never send a human to do a wolf's job cause it would be really really really be funny to watch. :p"; RectangleF displayRectangle = new RectangleF(0, 40, 200, 200); //GenericDefault, or new StringFormat() do a better job here then StringFormat.TypoGraphic //you also need both these format flags. StringFormat strFormat = StringFormat.GenericTypographic; strFormat.FormatFlags = StringFormatFlags.FitBlackBox | StringFormatFlags.NoClip | //vital or the drawn text will look shredded StringFormatFlags.MeasureTrailingSpaces; //so space in end of your text will also get measured CharacterRange[] ranges; Region[] charRegions; Color foreColor; Color backColor; for (int index = 0, i; index < text.Length; index += 32) { if (text.Length - index < 1) //if index becomes bigger then the text length break; else if (text.Length - index > 32) //if we're guarenteed 32 characters of bliss ranges = new CharacterRange[32]; else //we got less then 32 characters, but atleast 1 ranges = new CharacterRange[text.Length - index]; //get the character ranges for the 32 char-length or less segment of text we're at for (i = 0; i < ranges.Length; i++) ranges[i] = new CharacterRange(index + i, 1); //setup for measure strFormat.SetMeasurableCharacterRanges(ranges); //measure it charRegions = e.Graphics.MeasureCharacterRanges(text, this.Font, displayRectangle, strFormat); //draw text one character at a time for (i = 0; i < charRegions.Length; i++) { //figure out the colors of the text and background foreColor = RandomColor(); backColor = InvertColor(foreColor); foreColorSolidBrush.Color = foreColor; backColorSolidBrush.Color = backColor; //draw the background e.Graphics.FillRegion(backColorSolidBrush, charRegions[i]); //draw the text RectangleF bounds = charRegions[i].GetBounds(e.Graphics);e.Graphics.DrawString(text[index + i].ToString(), this.Font, foreColorSolidBrush, bounds, strFormat); } } } } } }
This is my solution. I thought I should share it as well. 这是我的解决方案。我想我也应该分享它。
I had no need for multi-line text but if you want that you should replace new RectangleF(0, 0, 1000, 1000) with something more suitable for you.
我不需要多行文本,但是如果你想的话,你应该更换新的 RectangleF(0, 0, 1000, 1000),会更适合你。
public static RectangleF[] MeasureString(Graphics graphics, Font font, String text)
{
int max = 32;
int length = text.Length;
RectangleF[] sizes = new RectangleF[length];
for (int j = 0; j < Math.Ceiling(length / (double)max); j++)
{
int max_f = length >= (j + 1) * max ? max : length - j * max;
CharacterRange[] ranges = new CharacterRange[max_f];
for (int i = 0; i < max_f; i++)
{
ranges[i] = new CharacterRange(i + j * max, 1);
}
StringFormat format = new StringFormat(StringFormatFlags.FitBlackBox | StringFormatFlags.NoClip | StringFormatFlags.MeasureTrailingSpaces);
format.SetMeasurableCharacterRanges(ranges);
Region[] regions = graphics.MeasureCharacterRanges(text, font, new RectangleF(0, 0, 1000, 1000), format);
for (int i = 0; i < max_f; i++)
{
RectangleF bounds = regions[i].GetBounds(graphics);
sizes[i + j * max] = bounds;
}
}
return sizes; }