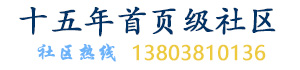
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。”需要全文内容也请联系孙老师。
The map is a template that uses a key to obtain a value.
Another issue is that you will want to use your own classes instead of data types, like int that has been used up to now. To create a class that is "template-ready", you must be ensure that the class contains certain member functions and operators. The basics are:
You would overload more operators as required in a specific template, for example, if you plan to have a class that is a key in a map you would have to overload relational operators. But that is another story.
// Program: Map Own Class // Purpose: To demonstrate a map of classes #include <string> #include <iostream> #include <vector> #include <map> using namespace std; class CStudent { public : int nStudentID; int nAge; public : // Default Constructor - Empty CStudent() { } // Full constructor CStudent(int nSID, int nA) { nStudentID=nSID; nAge=nA; } // Copy constructor CStudent(const CStudent& ob) { nStudentID=ob.nStudentID; nAge=ob.nAge; } // Overload = void operator = (const CStudent& ob) { nStudentID=ob.nStudentID; nAge=ob.nAge; } }; int main(int argc, char* argv[]) { map <string, CStudent> mapStudent; mapStudent["Joe Lennon"] = CStudent(103547, 22); mapStudent["Phil McCartney"] = CStudent(100723, 22); mapStudent["Raoul Starr"] = CStudent(107350, 24); mapStudent["Gordon Hamilton"] = CStudent(102330, 22); // Access via the name cout << "The Student number for Joe Lennon is " << (mapStudent["Joe Lennon"].nStudentID) << endl; return 0; }
To avoid an annoying error code in debug mode, use the following compiler pragma:
#pragma warning(disable: 4786)
Another gotcha is: you must make sure that the spaces are placed between your angle brackets and the name. This is because >> is the bit shift operator, so:
vector <list<int>> veclis;
will give an error. Instead, write it:
vector <list <int> > veclis;
to avoid compilation errors.