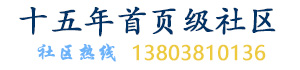
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。”需要全文内容也请联系孙老师。
This article discusses and implements 3-tier architecture using C# and a dummy customer using MS Access database. In this article I am trying to implement a small reusable component that maintains customers in 3-tier architecture. It shows how to add, update and find customer details.
To begin with I would like to discuss a little about the theoretical aspects of 3-tier architecture. I'll briefly go through what 3-tier architecture is and what are its advantages.
Three-tier (layer) is a client-server architecture in which the user interface, business process (business rules) and data storage and data access are developed and maintained as independent modules or most often on separate platforms. Basically, there are 3 layers, tier 1 (presentation tier, GUI tier), tier 2 (business objects, business logic tier) and tier 3 (data access tier). These tiers can be developed and tested separately.
What is the need for dividing the code in 3-tiers? Separation of the user interface from business logic and database access has many advantages. Some of the advantages are as follows:
This component has 3 tiers. Tier 1 or GUI tier with a form will be called FrmGUI, tier 2 or business logic will be called BOCustomer short for Bussniess Object Customer and finally the tier 3 or the data tier will be called DACustomer short for Data Access Customer. I have compiled all the 3 tiers in the same project for ease of work. I am including all the source code along with the MS Access database that is used to test this project in the included zip file.
This is a chunk of code from the user interface. I am only including the functions that are used to call the middle tier or the business logic layer.
I am keeping a reference to business logic layer as BOCustomer.
//This function get the details from the user via GUI //tier and calls the Add method of business logic layer. private void cmdAdd_Click(object sender, System.EventArgs e) { try { cus = new BOCustomer(); cus.cusID=txtID.Text.ToString(); cus.LName = txtLName.Text.ToString(); cus.FName = txtFName.Text.ToString(); cus.Tel= txtTel.Text.ToString(); cus.Address = txtAddress.Text.ToString(); cus.Add(); } catch(Exception err) { MessageBox.Show(err.Message.ToString()); } } //This function gets the ID from the user and finds the //customer details and return the details in the form of //a dataset via busniss object layer. Then it loops through //the content of the dataset and fills the controls. private void cmdFind_Click(object sender, System.EventArgs e) { try { String cusID = txtID.Text.ToString(); BOCustomer thisCus = new BOCustomer(); DataSet ds = thisCus.Find(cusID); DataRow row; row = ds.Tables[0].Rows[0]; //via looping foreach(DataRow rows in ds.Tables[0].Rows ) { txtFName.Text = rows["CUS_F_NAME"].ToString(); txtLName.Text = rows["CUS_L_NAME"].ToString(); txtAddress.Text = rows["CUS_ADDRESS"].ToString(); txtTel.Text = rows["CUS_TEL"].ToString(); } } catch (Exception err) { MessageBox.Show(err.Message.ToString()); } } //this function used to update the customer details. private void cmdUpdate_Click(object sender, System.EventArgs e) { try { cus = new BOCustomer(); cus.cusID=txtID.Text.ToString(); cus.LName = txtLName.Text.ToString(); cus.FName = txtFName.Text.ToString(); cus.Tel= txtTel.Text.ToString(); cus.Address = txtAddress.Text.ToString(); cus.Update(); } catch(Exception err) { MessageBox.Show(err.Message.ToString()); } }