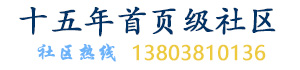
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。”需要全文内容也请联系孙老师。
//this function can be represented //as a class with properties and functions inside it.这个函数能以带有内置属性和函数的类的形式重新编写。 function Cat() { this.name = "kitty" this.type = "big"; this.Details = function () { alert(this.name +" " +this.type); } } //This method is shared within all objects //Prototype is used to define the template of the object and used //to implement inheritance. Reference to objects and arrays added to //the prototype object are shared between all the instances. //functions defined in prototype are also shared between objects and it //saves a little memory but are a little slow to call as compared to //functions defined in constructor //another way to write Cat.prototype.speak = function().... 下面方法是所有对象共享的。Prototype用于定义对象的模板且用于实现继承。对添加到原型里的对象和数组的引用被所有实例共享。在原型里定义的函数同 样在对象之间共享且它会节省些内存,但是在调用上比在构造函数里写的函数会慢些。 另外的写法有Cat.prototype.speak = function() Cat.prototype={ speak : function() { alert('meaaoooww'); } } //Static Function on Cat Object Cat对象的静态函数 Cat.StaicFunc = function() { //this.name or any other property is not accessible //because it is a static method this.name或其它别的属性是不可访问的,因为它们是静态方法 alert("static function called"); } //object of class;类的对象 var cat = new Cat(); cat.speak(); cat.Details(); Cat.StaicFunc(); Inheitance can be implemented in JavaScript as function WildCat() { Cat.call(this); //Calling the base constructor调用基类的构造函数 } WildCat.prototype = new Cat(); //Overriding the base function speak覆盖基类的函数speak WildCat.proptotype.speak = function() { alert('this is wild cat..Beware'); }
A quicker way to create an object is:创建对象的快速方法是:
var team = {name:'', members:[], count:function() { return members.length }}
The above object is created using the JSON format. To learn more about the JSON format, "Google" for it :-).上面的对象以JSON格式创建。
JavaScript in the AJAX Library在AJAX库里的JavaScriptType.registerNamespace('Sample'); Sample.Vehicle = function () { this._name; this._type; } Sample.Vehicle.prototype = { get_name : function() { return this._name; }, set_name : function (value) { this._name = value; }, get_type : function() { return this._type; }, set_type : function(value) { this._type = value; }, Honk : function() { alert('Loud noise coming...cant you hear that'); } } Sample.Vehicle.registerClass('Sample.Vehicle');
In the above example, you just need to call registerNamespace and registerClass to register your namespaces and classes with the AJAX Library. The above class has two properties and a function. The get_ and set_ format should be used to get and set the values of properties.上例中,你只需要调用registerNamespace和registerClass来注册你的命名空间和类到AJAX库里即可。上面类里有2个属性和一个函数。get_和set_形式在获取和设置属性时使用。
The format for registerClass is something like: registerClass的形式如下:
NameofClass.registerClass('NameofClass', BaseClass, InterfacesImplemented)
An interface can be defined as:接口可按如下形式定义:
Sample.IDrive = function() { throw Error.notImplemented(); } Sample.IDrive.prototype = { Drive : function () { throw Error.notImplemented(); } } Sample.IDrive.registerInterface('Sample.IDrive');To inherit a class from the above Vehicle class and implement the interface为了从上面Vehicle类继承和实现接口:
Sample.Honda = function() { Sample.Vehicle.initializeBase(this); //if base class takes arguments in constructor //then they can also be passed //Sample.Vehicle.initializeBase(this, [arg1,arg2,...]); } Sample.Honda.prototype = { Honk: function() { alert(this._name + ' honking ...'); //To call base method //Sample.Honda.callBaseMethod(this, 'Test',[arg1,arg2,…]); } , Drive : function() { alert('Driving...'); } } Sample.Honda.registerClass('Sample.Honda',Sample.Vehicle, Sample.IDrive);
To add/remove an event, use add_eventname/remove_eventname. For example:为了添加/删除事件,使用add_eventname/remove_eventname.比如: