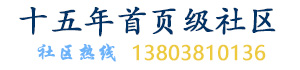
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。需要全文内容也请联系孙老师。
Recently, I needed to make an ActiveX component in C++ to be used in my C# .NET program. However, I could not find any useful tutorial which could guide me through the dark world of C++ MFC. I started to do some investigation on how things work in C++/MFC and how C# reacts to importing an ActiveX component. If you like to know how to program in C++ or use the MFC library, then stop reading this tutorial. This tutorial is just a guide on how to create a decent ActiveX component that .NET environment can easily convert.
最近,我需要让我的C#程序中使用C ++中的ActiveX组件。但是,我找不到任何有用的教程它可以引导我通过C ++ MFC的黑暗的世界。我开始做一些调查,在c++ / MFC的工作原理和导入一个ActiveX组件时c#如何反应。如果你想知道如何在c++编制程序或使用MFC库,那么停止阅读本教程。本教程是一个如何创建一个像样的.net环境可以很容易地转换的ActiveX组件。
More information about ActiveX can be found here. ActiveX的更多信息可以在这里找到。
The first step we need to do is create the C++ MFC project: 我们需要做的第一步是创建c++ MFC项目:
Give the project a name, and click on "OK", and later on "Finish". The funny thing about ActiveX is that you haven't yet coded anything, but the project is already filled with a lot of code. The ActiveX project uses MFC in a shared DLL. Change it into Static Library. Open the properties of the project, and change the "Use MFC" option into "Use MFC in a Static Library".
给项目起一个名字,并点击“OK”,然后“完成”。关于ActiveX有趣的事情是你还没有编写任何东西,但该项目已经充满了大量的代码。该ActiveX项目在DLL共享中使用MFC。改变成静态库。打开项目属性,更改“使用MFC”选项为“使用MFC在静态库”。
So, why is this step required? Registering the ActiveX component on a different machine where no Visual Studio has been installed will fail. The ActiveX component will miss the MFC DLLs. If this is not clear yet, just play around with the Depends.Exe located here: C:\Program Files\Microsoft Visual Studio 8\Common7\Tools\Bin.
那么,为什么需要这一步?在没有安装Visual Studio的机器上注册ActiveX组件将会失败。该ActiveX组件将错过MFC DLL文件。如果这还不清楚,只是摆弄位于:C:\Program Files\Microsoft Visual Studio 8 \ Common7\Tools\Bin的Depends.Exe.。
The ActiveX project creates five header files and six source files. These header/source files are required for this part: ActiveX项目创建五个头文件和六个源文件。这些标题/源文件需要这部分:
CppActiveXCtrl.cpp CppActiveXCtrl.h CppActiveX.idl
First, create a method in the CppActiveXCtrl.h file, just where the AboutBox method is declared. 首先,在CppActiveXCtrl.h文件中创建一个方法,只是AboutBox方法的声明 。
afx_msg void AboutBox(); afx_msg LONG TestMyMethod( LONG param1, LONG param2, BSTR param3 );
Create the method in the source file of this header. Return some value (it's just an example). 创建源文件标题的方法。返回某个值(这只是一个例子)。
LONG CCppActiveXCtrl::TestMyMethod( LONG param1, LONG param2, BSTR param3 )
{
return -5;
}
Every input method which could be used must have a unique identifier. So, create some unique identifiers. Be careful not to use Windows IDs which are declared in olectl.h. 可以使用的每个输入方法必须有一个惟一的标识符。所以,创造一些独特的标识符。小心不要使用在olectl.h声明的Windows标识。
Create this const in the header files CppActiveXCtrl.h and CppActiveX.idl [Note: you can also make a global ID for this (just whatever you like)]: 在CppActiveXCtrl.h和CppActiveX.idl中创建这个头文件[注:你也可以做一个全局ID(无论你喜欢什么):
static const int DISPID_TEST_METHOD = 1025314;or (select any style you like): 或(选择任何你喜欢的风格):
#define DISPID_TEST_METHOD (1025314)
Go to CppActiveX.idl and implement the following code in the dispinterface of the ActiveX. You can also find the AboutBox method here. Use the ID for your method as declared before. Every method should have its unique identifier.
转到C++ ActiveX.idl并在ActiveX的调度接口实现下面的代码。你也可以在这里找到AboutBox方法。使用ID的方法声明之前。每个方法都应该有其独特的标识符。
dispinterface _DCppActiveX { properties: methods: [id(DISPID_ABOUTBOX)] void AboutBox(); [id(DISPID_TEST_METHOD)] LONG TestMyMethod(LONG param1, LONG param2, BSTR param3);
Go back to the CppActiveXCtrl.cpp file. Go to the Dispatch map part of this file. Add the following for your TestMyMethod. See this example:
返回CppActiveXCtrl.cpp文件。去调度文件内的映射。添加以下为你准备的测试方法。看这个例子:
BEGIN_DISPATCH_MAP(CCppActiveXCtrl, COleControl) DISP_FUNCTION_ID(CCppActiveXCtrl, "AboutBox", DISPID_ABOUTBOX, AboutBox, VT_EMPTY, VTS_NONE) DISP_FUNCTION_ID(CCppActiveXCtrl, "TestMyMethod", DISPID_TEST_METHOD, TestMyMethod, VT_I4, VTS_I4 VTS_I4 VTS_BSTR) END_DISPATCH_MAP()
Note: make sure you use the correct type of input. Otherwise, this could lead to some nasty exceptions. 注意:确保您使用正确的输入类型。否则,这可能会导致一些严重的异常。
OK, one method and one event should make it clear. Go to the Class View of Visual Studio, and select CCppActiveXCtrl. Hover on Add, and add this event: 好了,一个方法和一个事件应该弄清楚。转到Visual Studio的类视图,并选择CCppActiveXCtrl。鼠标箭头放在Add菜单上,再点Add Event:
Add the following event with some default parameters. Just pick some.... 添加以下事件与一些默认的参数。挑选一些....
Now, compile the project. 现在,编译项目。
Create a C# "Windows Application" project. I'm not going to explain how to create a C# .NET project here. 创建一个c#“Windows应用程序”项目。在这里我不打算解释如何创建一个c#.NET项目。
During the compilation of the ActiveX project, the ActiveX component "ocx" file will be registered automatically. If not, use the command line: 在ActiveX项目编译的时候,ActiveX组件“ocx文件将被自动注册。如果没有,使用命令行:
regsvr32 /i CppActiveX.ocx
Select the main form, and with the right mouse button, add an item. 选择主要形式,然后用鼠标右键,添加一个项目。
Select the COM components, and find the CppActiveX control. Press "OK" to add it to the toolbox. 选择COM组件,并找到CppActiveX控件。按“确定”将其添加到工具箱。
Drag the added ActiveX control to your form. Note: if you change the input methods for your ActiveX project, make sure you delete the obj directory as well as the bin directory. 拖动ActiveX控件添加到表单。注意:如果你改变ActiveX项目的输入方法,确保你删除obj目录以及bin目录。
Interop.CppActiveXLib.dll AxInterop.CppActiveXLib.dllHere is an example of the code when using the OCX file: 这是使用OCX文件一个例子的代码:
public Form1() { InitializeComponent(); int k = axCppActiveX1.TestMyMthod(4,4,"Hi"); axCppActiveX1.EventHandlerTest += new AxCppActiveXLib._DCppActiveXEvents_EventHandlerTestEventHandler( axCppActiveX1_EventHandlerTest); }private void axCppActiveX1_EventHandlerTest( object sender, _DCppActiveXEvents_EventHandlerTestEvent e ) { int age = e.age; string name = e.name; string surname = e.surname;