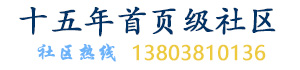
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
I was browsing through some folders the other day when I stumbled into the Cookies folder. I was shocked, to say the least, to see several thousand files in there, some of which were nearly three years old. Thinking that something must be wrong with IE's cookie-related settings, I checked IE's properties and everything looked as it should. I clicked on the Delete Cookies button and was presented with a "Delete all cookies in the Temporary Internet Files folder?" message. I responded in the affirmative and then went to check the Cookies folder. It was untouched! All other temporary files had been deleted, however. At this point, I was not sure if the Cookies folder was a special case, or if I was just missing something obvious (it happens). I tried this same thing on a few other machines and got mixed results. On some of them, the Cookies folder was emptied. On others, the folder remained untouched.作者偶尔看到Cookies目录,目录里大量文件个数让他震惊,还有些超过3年的文件还在。用IE配置里的“删除 Cookies”按钮,当时没起作用,作者又找了些机器试验,有些行,有些不行。
Back when I was doing Web development, I seem to remember an Expires attribute of the Cookies collection that was used to set the date on which the cookie would expire. I left that company before we ever got around to verifying whether cookies did all they needed to or not. Maybe, I'm incorrect in thinking that they would automatically get cleaned up when the date indicated by the Expires attribute had passed. Instead, maybe it is simply an indicator to the Web page that the cookie is too old to be used and that another should be created. I welcome any input on this mystery.当进行Web开发时,Cookies的Expires属性设置超过时间。当Expires属性规定的时间到时,Cookies内容会被自动删除,这个思考结果我不敢确定。可能,它只是个指示器,指示Web页面说cookie太旧了,需要创建新的。
That said, I thought I would put together an article on what it would take to automatically clean out the Cookies folder of any old/unused files. The following sections are a brief discussion of that endeavor. Feel free to change the code to fit your needs (e.g., more options, more flexibility, more error checking).所以我写此文讨论自动删除Cookies目录下文件或目录。下节是简短的讨论。本文代码可以自由扩充(比如更多选项,更灵活,更多错误检查)
Please note that I did not create this article/utility with the intent of replacing something that IE does internally. It was merely an attempt at addressing what appears to be an inconsistency in how IE manages its Cookies folder. If IE cleans up your Cookies folder with no troubles, this article is not intended for you. If, however, you find that IE does not clean up your Cookies folder, you might just find this article helpful. Enjoy!非替换IE功能。只是关注于IE处理Cookies目录的不确定性。
So, where is the Cookie jar folder anyhow? The easiest way to find out is with the SHGetFolderPath() function.用SHGetFolderPath()函数可以查找到。
// call GetBuffer() once instead of potentially calling it twice below
CString strCookiesPath;
LPTSTR lpszPath = strCookiesPath.GetBuffer(MAX_PATH);
// where is the 'jar' of cookies?
HRESULT hResult = SHGetFolderPath(NULL, CSIDL_COOKIES,
NULL, SHGFP_TYPE_CURRENT, lpszPath);
if (FAILED(hResult))
hResult = SHGetFolderPath(NULL, CSIDL_COOKIES,
NULL, SHGFP_TYPE_DEFAULT, lpszPath);
strCookiesPath.ReleaseBuffer();
At this point, strCookiesPath should contain something like "C:\Documents and Settings\<user>\Cookies". To begin searching this folder, all we need to do is tack on a backslash and a pair of wildcard characters, and we're off! Prior to MFC, I would have employed code like the following to do this:代码运行后,strCookiesPath变量应该包含如下"C:\Documents and Settings\<user>\Cookies"。为了开始搜索此目录,我们需要添加反斜杠。
char szPath[256] = "C:\Documents and Settings\<user>\Cookies";
if (szPath[strlen(szPath)] != '\\')
strcat(szPath, "\\");
This worked as intended, but when I started using MFC back in 1993, I switched to CString objects instead. This resulted in:也可以用MFC里的CString类。
strCookiesPath = "C:\Documents and Settings\<user>\Cookies";
if (strCookiesPath.Right(1) != "\\")
strCookiesPath += "\\";
This was a little bit cleaner but then the problem of localization came up. Hard-coding the backslash character could invite trouble. It has to do with the Right() method. In DBCS languages (e.g., Japanese), this is a common bug because if the last character in the string is double-byte, and the trail byte is 0x5C (the value of backslash), the code will mistakenly think a backslash is already there (thanks Michael Dunn). To address that, I used PathAddBackslash() like:上面代码比较清晰,但本地化问题会浮出水面。硬编码反斜杠会导致问题。必须用Right()方法才能解决。在DBCS语言里(比如日文),是常见bug,因为最后字符是双字节,最后字节是0x5C(反斜杠编码),代码会误认为反斜杠已经有了。为了解决它,使用了PathAddBackslash()
// add a backslash if necessary
PathAddBackslash(strCookiesPath.GetBuffer(MAX_PATH));
strCookiesPath.ReleaseBuffer();
Our string now contains a trailing backslash. Now we are ready to search (or process) the Cookies folder. To do this, I simply used a CFileFind object. Since the whole premise of this article was to find cookies that had not been accessed in a certain number of days, we simply need to compare the cookie's last-accessed date with some other user-configured date (i.e., cutoff date). If the cookie is old enough, it is added to a list of cookies to be removed, or displayed, later. I am using Michael Dunn's CShellFileOp class for this. This is a nice wrapper around the SHFileOperation() function.使用CFileFind来搜索目录。本文关注数天内没使用的cookies,我们只需要简单地比较cookie的最后访问日期,把它和一些用户配置日期(截止日期)。如果cookie足够老,会添加到删除列表里或随后显示。我使用了Michael Dunn's的CShellFileOp类实现此功能。它对SHFileOperation()函数封装的很棒。
CShellFileOp shfo;
CTime dateFile,
dateCutoff;
CFileFind fileFind;
BOOL bFound = fileFind.FindFile(strCookiesPath + _T("*.*"));
while (TRUE == bFound)
{
bFound = fileFind.FindNextFile();
if (! fileFind.IsDirectory())
{
// if the file was last written to before the cutoff date,
// add it to the list of files to be removed
fileFind.GetLastWriteTime(dateFile);
if (CTime(dateFile.GetTime()) < dateCutoff)
shfo.AddSourceFile(fileFind.GetFilePath());
}
}
We now have a list of cookies that can either be deleted, or output to the screen (maybe you want to see the cookies that would be deleted). To show the cookies, just iterate through the list. CShellFileOp provides a method, GetSourceFileList(), to access this list.得到列表,就可让列表删除或显示出来。通过遍历列表,CShellFileOp提供了函数GetSourceFileList()
const CStringList &listSourceFiles = shfo.GetSourceFileList();
POSITION pos;
CString strFilename;
pos = listSourceFiles.GetHeadPosition();
while (NULL != pos)
{
strFilename = listSourceFiles.GetNext(pos);
cout << (LPCTSTR) strFilename << endl;
}
If however, we actually wanted to delete the cookies, we would just use the CShellFileOp::Go() method for this. For more on how to use this class, including how to move cookies to the Recycle Bin instead of deleting them, consult Michael's page.
The cutoff date can be calculated by simply constructing a CTimeSpan object and subtracting it from the current date. For example, if you wanted to delete cookies that had not been accessed within the last 15 days, you would specify a cutoff date of:构造CTimeSpan对象,用当前时间减去构造时间,就可计算出来。比如你想删除15天内没有用到的cookies项,用如下代码设定:
Now, if the cookie's last-accessed date is older than the cutoff date, it will get deleted.如果cookies项最后访问时间比截止时间早,就会被删除。
Using the utility, aptly named cookiemonster, in its current state, five command-line switches are supported. The handling of these is done via Paul DiLascia's CCommandLineInfoEx class. Here is a synopsis of each:使用命名为cookiemonster的工具,用好它的5个命令行选项。命令行处理通过CCommandLineInfoEx类来实现。下面是命令行选项介绍:
/days ## |
Number of days since cookie was last accessed.从最后访问时间算出的天数 |
|
/list |
Show cookies that would be deleted. Cookies are not affected if this is used.显示要被删除的cookies项。如果正在使用,不受影响。 |
|
/verbose |
Show details of what the program is doing.显示细节 |
|
/recycle |
Move cookies to the Recycle Bin. Ignored if /list is used.移动到回收站里。如果/list使用,则此项被忽略。 |
|
/? |
Show the help screen.显示帮助。 |
Issuing cookiemonster /days 2 /verbose /recycle at a command prompt, which could just as easily be a scheduled task, yielded the following result on my machine:
Deleting cookies last accessed before 10/19/04.
Cookies will be moved to the Recycle Bin.
Deleting cookies from C:\Documents and Settings\davidc\Cookies.
File: davidc@arstechnica[2].txt.
...Date: 10/21/04
File: davidc@arvest[2].txt
...Date: 10/19/04
...Added
File: davidc@ask[2].txt
...Date: 10/20/04
File: davidc@bbc.co[1].txt
...Date: 10/19/04
...Added
File: davidc@cardeals[2].txt
...Date: 10/20/04
File: davidc@celebritytrivia[1].txt
...Date: 10/20/04
File: davidc@channelintelligence[2].txt
...Date: 10/21/04
<balance of output deleted for brevity>
Note that two files were added to the list, while five were not because their last-accessed date was newer than the cutoff date.