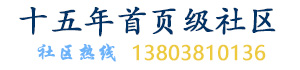
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
The requirements for an HTML form to be able to upload files are very simple: you have to use multipart/form-data encryption and you have to use method of post.
能上传文件的HTML表单的要求,非常简单:你必须使用 multipart / form-data加密,且必须使用POST方法。
<form id="Form1" method="post" runat="server" enctype="multipart/form-data">
HTML input control that is used to upload file has a simple type of file.
HTML输入控件可用来上传文件,它有一个简单的文件类型。
<input id="filMyFile" type="file" runat="server">
This is all that needs to be done to an HTML form for a client to be able to submit a file to your ASP.NET application.
这是所有需要做的到HTML表单的客户端能够将文件提交到你的ASP.NET应用程序。
<INPUT TYPE="file" corresponds to the System.Web.UI.HtmlControls.HtmlInputFile type of object on the server side. So if you, like myself, are using a CodeBehind architecture for your page you will have a member field definition similar to this in your class:
<INPUT TYPE="file"对应服务器端的System.Web.UI.HtmlControls.HtmlInputFile类型的对象。所以,如果你像我一样,用的是代码隐藏架构为您的网页,你会在你的类类似下面的成员字段的定义:
protected HtmlInputFile filMyFile;
HtmlInputFile classes have a few different properties, but the only one that really concerns us here isPostedFile. This property will tell us all we need to know about the file that had been uploaded to our server. The PostedFile property is null if no file was submitted by a client. So, we can simply check whether a file was sent by doing something like this:
HtmlInputFile类有几个不同的属性,但只有一个真正关系到我们是PostedFile。此属性将告诉我们一切,我们需要了解已被上传到我们的服务器上的文件。如果没有文件提交由客户端的PostedFile属性为null。所以,我们可以简单地检查文件是否被送往做这样的事情:
if( filMyFile.PostedFile != null ) { // File was sent } else { // No file }
The PostedFile property will contain a valid System.Web.HttpPostedFile object if file indeed was uploaded.HttpPostedFile provides us with 4 properties:
该PostedFile属性将包含一个有效的System.Web.HttpPostedFile对象,如果文件确实是uploaded.HttpPostedFile为我们提供了4个属性:
让我们来看看该文件的大小:
// Get a reference to PostedFile object HttpPostedFile myFile = filMyFile.PostedFile; // Get size of uploaded file int nFileLen = myFile.ContentLength;
Now that we know the size, we can move on to retrieving the data. First, we need to allocate a byte array to store the data:
现在我们知道的大小,我们可以继续检索数据。首先,我们需要分配一个字节数组来存储数据:
// Allocate a buffer for reading of the file byte[] myData = new byte[nFileLen];
Next, we can read the uploaded file in our buffer using the InputStream object:
接下来,我们可以阅读使用InputStream对象我们的缓冲区上传的文件:
// Read uploaded file from the Stream myFile.InputStream.Read(myData, 0, nFileLen);
At this point, we have successfully retrieved the uploaded file into a byte array called myData. What to do with it next depends largely on the requirements of your application. I will show both saving the file to hard drive and to a database in the next sections.
在这一点上,我们已经成功地获取上传的文件到名为myData的字节数组。怎么用它做下一很大程度上取决于应用程序的需求。我会同时显示将文件保存到硬盘驱动器,并在接下来的章节中的数据库。
I wrote a simple function for my demo project that stores files to a disk:
我写了一个简单的函数为我演示项目存储磁盘文件:
// Writes file to current folder private void WriteToFile(string strPath, ref byte[] Buffer) { // Create a file FileStream newFile = new FileStream(strPath, FileMode.Create); // Write data to the file newFile.Write(Buffer, 0, Buffer.Length); // Close file newFile.Close(); }
I pass a full path of where I want a file to be stored and reference to a buffer with file data to this function. It uses the System.IO.FileStream object to write a buffer to a disk. This very simplistic 3 lines of code approach will work in most cases. A couple of considerations here are: getting the filename of the uploaded file and the security. Since the FileName property of PostedFile is a full path to the uploaded file on a client's computer, we will probably want to use only the filename portion of that path. Instead of using some parsing techniques to look for backslashes and things like that, we can use a very convenient little utility class: System.IO.Path.
我想传递一个完整路径的文件存储和引用与文件数据缓冲区,这个函数。它使用先。文件流对象缓冲区写入磁盘。这个非常简单的3行代码的方法将在大多数情况下工作。几个因素是:上传文件的文件名和安全。由于PostedFile的文件名属性是一个完整路径上传文件在客户的电脑上,我们可能会想要使用唯一的文件名部分路径。而不是使用一些解析技术寻找反斜杠之类的东西,我们可以使用一个非常方便的小工具类:System.IO.Path。
string strFilename = Path.GetFileName(myFile.FileName);
Security is another matter. In my demo project, I store files in the same folder where the project is executed. In order for that to work, the ASPNET account (the account that is used to execute ASP.NET processes) has to have Write permissions on that folder. By default it does not, so you need to right-click the folder, go to the security tab and add the ASP.NET account to the list. Then grant write permissions to that account by checking the Write checkbox and click OK.
安全是另一个问题。在我的演示项目,我存储文件在同一文件夹项目执行。为了工作,ASPNET帐户(帐户,用于执行ASP。净过程)有写权限,文件夹。默认情况下它不,所以你需要右键单击文件夹,去security选项卡添加ASP。网络账户列表。然后写权限授予账户通过检查写复选框并单击OK。
The following function is used in my demo project to store uploaded files in the database:
下列函数中使用我的演示项目数据库中存储上传文件:
// Writes file to the database private int WriteToDB(string strName, string strType, ref byte[] Buffer) { int nFileID = 0; // Create connection OleDbConnection dbConn = new OleDbConnection(GetConnectionString()); // Create Adapter OleDbDataAdapter dbAdapt = new OleDbDataAdapter("SELECT * FROM tblFile", dbConn); // We need this to get an ID back from the database dbAdapt.MissingSchemaAction = MissingSchemaAction.AddWithKey; // Create and initialize CommandBuilder OleDbCommandBuilder dbCB = new OleDbCommandBuilder(dbAdapt); // Open Connection dbConn.Open(); // New DataSet DataSet dbSet = new DataSet(); // Populate DataSet with data dbAdapt.Fill(dbSet, "tblFile"); // Get reference to our table DataTable dbTable = dbSet.Tables["tblFile"]; // Create new row DataRow dbRow = dbTable.NewRow(); // Store data in the row dbRow["FileName"] = strName; dbRow["FileSize"] = Buffer.Length; dbRow["ContentType"] = strType; dbRow["FileData"] = Buffer; // Add row back to table dbTable.Rows.Add(dbRow); // Update data source dbAdapt.Update(dbSet, "tblFile"); // Get newFileID if( !dbRow.IsNull("FileID") ) nFileID = (int)dbRow["FileID"]; // Close connection dbConn.Close(); // Return FileID return nFileID; }
The Access database that I used in the demo project has only one table tblFile that has 5 fields defined as follows:
FileID – autonumber Filename – text 255 FileSize - long integer ContentType – text 100 FileData – OLE Object
The code in the above function is very straightforward. I create OleDbConnection, OleDbDataAdapter andDataSet objects and then append a row to the only table in DataSet. The important thing to note here is that the OleDbCommandBuilder object needs to be created and initialized with a reference to theOleDbDataAdapter object to build the insert query for us automatically. Also, if you would like to retrieve the newly assigned FileID of the file you have just stored in the database, you need to make sure you set theMissingSchemaAction property of Adapter to MissingSchemaAction.AddWithKey. That assures that the Primary Key/autonumber FileID field will be populated with the new ID when you call the Update method of yourDataAdapter.
The local ASP.NET account has to have Write permissions to a database file in order for your code to succeed. If your file is placed anywhere under wwwroot, you will need to manually give the database file all the necessary permissions before running my demo project.
我用于演示项目的访问数据库只有一个表tblFile有5个字段定义如下:
FileID – autonumber Filename – text 255 FileSize - long integer ContentType – text 100 FileData – OLE Object
上面的函数中的代码非常简单。我创建OleDbConnection,OleDbDataAdapter andDataSet对象,然后添加一行唯一的表数据集。这里值得注意的一件重要事情是,OleDbCommandBuilder对象需要创建和初始化引用theOleDbDataAdapter对象自动为我们构建插入查询。同样,如果你想检索新分配的文件标识的文件你存储在数据库中,你需要确保你设置theMissingSchemaAction属性MissingSchemaAction.AddWithKey适配器。保证主键/ autonumber文件标识字段将填充新的ID当你叫yourDataAdapter的更新方法。
当地的ASP。网帐户必须有写权限数据库文件代码为了成功。wwwroot下如果你的文件放置在任何地方,您将需要手动把数据库文件在运行我的演示项目之前所有必要的权限。
I used the same ASPX page to read and return file data out of the database in my demo project. I check for theFileID parameter being passed to a page. If it was passed, I know that I need to return a file rather than process the user's input:
我用相同的ASPX页面读取并返回文件从数据库中数据在我的演示项目。我检查theFileID参数被传递给一个页面。如果是过去了,我知道我需要返回一个文件而不是处理用户的输入:
private void Page_Load(object sender, System.EventArgs e) { // Check if FileID was passed to this page as a parameter if( Request.QueryString["FileID"] != null ) { // Get the file out of database and return it to requesting client ShowTheFile(Convert.ToInt32(Request.QueryString["FileID"])); } }
The ShowTheFile function does all the work here:
ShowTheFile函数做所有的工作:
// Read file out of the database and returns it to client private void ShowTheFile(int FileID) { // Define SQL select statement string SQL = "SELECT FileSize, FileData, ContentType FROM tblFile WHERE FileID = " + FileID.ToString(); // Create Connection object OleDbConnection dbConn = new OleDbConnection(GetConnectionString()); // Create Command Object OleDbCommand dbComm = new OleDbCommand(SQL, dbConn); // Open Connection dbConn.Open(); // Execute command and receive DataReader OleDbDataReader dbRead = dbComm.ExecuteReader(); // Read row dbRead.Read(); // Clear Response buffer Response.Clear(); // Set ContentType to the ContentType of our file Response.ContentType = (string)dbRead["ContentType"]; // Write data out of database into Output Stream Response.OutputStream.Write((byte[])dbRead["FileData"], 0, (int)dbRead["FileSize"]); // Close database connection dbConn.Close(); // End the page Response.End(); }
I am using OleDbConnection, OleDbCommand and OleDbDataReader objects to retrieve data. The next step is to clear the Response output buffer to make sure that no other information is being sent to the client besides ourfile data. That will only work if buffering of your pages is turned on (default in ASP.NET). I set the ContentTypeproperty of the Response object to the content type of our file. Then I write file data into the Output stream of the Response object. The important thing here is to call Response.End() at the end to prevent farther processing of this page. We are done here and we do not want for other code to execute.
我用OleDbConnection,OleDbCommand OleDbDataReader对象检索数据。下一步是明确响应输出缓冲区,以确保没有其他信息被发送到客户端除了ourfile数据。只会工作,如果缓冲页面打开在ASP.NET(默认)。我设置的ContentTypeproperty响应对象的内容类型文件。然后我文件数据写入输出流的响应对象。这里重要的是调用Response.End()结束时,以防止进一步处理这个页面。我们在这里做的,我们不希望其他代码执行。
重点
Because my demo project writes files to disk or data to an Access database, you need to make sure that security permissions are set properly on folders and files where you want to write. Beta versions of .NET used the Systemaccount to execute ASP.NET processes and it had access to everything on your computer. However, the final release of .NET runs all ASP.NET processes under a local ASP.NET account. That account needs to have Write permissions to all the folders and files that are being used to write data.
因为我的演示项目写文件到磁盘或数据访问数据库,您需要确保安全权限设置正确的文件夹和文件你想写的地方。Beta版本的。网使用帐号执行ASP.净的过程和获得所有在您的计算机上。然而,最终释放。净运行所有ASP。净过程在一个当地的ASP。网络账户。账户需要有写权限的所有文件夹和文件被用于写入数据。
In order to make my demo project work under the .NET release version, you need to place it in a folder (anywhere under wwwroot) and set the permissions of that folder as follows: Right-Click the folder, go to Properties, Security tab, make sure the local ASPNET account has Read and Write permissions on that folder. If not, Add local ASPNET account to the list and give it read and write permissions. Click OK.
为了使我的演示项目能在.NET平台执行。你需要把它放在一个文件夹(在wwwroot下的任何子文件夹)和设置该文件夹的权限如下:右键单击文件夹,属性,安全选项卡中,确保当地ASPNET账户已读和写权限。如果不是,将本地ASPNET帐户添加到列表,并给定读和写权限。单击OK。