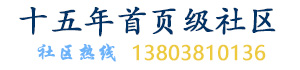
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明出处:锐英源,www.wisestudy.cn,孙老师作品,联系电话13803810136。
#include "Curve.h"
class myCurve : public CCurve
{
....
};
//cpp file
#include "myCurve.h"
CPoint3D myCurve::PointAtPara(double upar)
{
double x, y, z;
x = 2*sin(uPar)......
y = .......
z = .....
CPoint3D P(x,y,z);
return P;
}
CDocument相关位置添加如下代码...
#include "myCurve.h"
void CMyProjDoc::OnCurve()
{
myCurve crv(...);
CGLCurve* myglCurve = new CGLCurve(&crv)
dContext->Display(myglCurve);
delete myglCurve;
}
Now dContext is the display context object (CGLDisplayContext) that manages all the display functions, so this is created in the constructor of the document. Similarly for surfaces also. There are currently almost all the general curves and surfaces implemented. Curves are: line, circle, ellipse, parabola, hyperbola, Bezier and bspline. Surfaces are: plane, cylinder, cone, sphere, torus, extruded, revolved, ruled and pipe.现在dContext是显示用的上下文对象(CGLDisplayContext),它管理所有的显示功能,所以它要在文档的构造函数里创建。面的处理类似。目前几乎所有的曲线和曲面都实现过了。曲线有:直线、圆、椭圆、抛物线、双曲线、Bezier和B样条。表面有:平面、圆柱、圆锥、球体、圆环、挤压突出、旋转、嵌线和管道。
The display of all curves are managed by the CGLCurve class and that of surfaces are by the CGLSurface class. Points and other geometric helpers like axes, coordinate systems etc. are also modeled. This is implemented in non interactive mode for demo. You can modify the source to make an interactive application. CGLCurve类管理所有曲线显示,CGLSurface类管理所有表面显示。点和其他几何助手,比如像轴、坐标系统等也进行了模型化处理。本例以非交互模式实现,只用于演示。你可以修改源代码使它成为一个交互式应用程序。
There are some modifications, dated 23/2/2003, made in the CGLView class which is the basic class for OpenGL viewing. Earlier it was derived from CView and the CCadSurfView class was derived from CGLView. The OpenGL manipulation methods were called from the CCadSurfView class's methods. e.g. mouse implementations etc. Like this: CGLView::RotateView(). Some how, I felt that there is no isolation for the OpenGL view. Hence with a better Object Oriented approach, I made the CGLView class, an independent class and the constructor of the class is passed with pointer to CWnd. The private data member in CGLView is the pointer to the CWnd which is initialized with OpenGL settings in the c'tor. Now an object of this CGLView is private member for CCadSurfView class which is dynamically initialized in the OnCreate() method of CCadSurfView and is deleted in OnDestroy() method. Now the methods are called using the object of the CGLView class instead of calling it directly as base class methods.--Like this: 在23/2/2003做了些修改,是对 CGLView类进行的处理,这是基本的OpenGL视图类。此前它派生自CView,CCadSurfView类派生自CGLView。CCadSurfView类的方法里调用了OpenGL的操作,例如鼠标相关的消息处理函数里等等,像CGLView :: RotateView()里也有 。某种程度上,我觉得是没有为OpenGL视图进行隔离。这样,提供了更好的面向对象的方法,我完成了独立的CGLView类,它的构造函数里有CWnd类指针参数。CGLView类的私有数据成员是CWnd的指针,这个CWnd在构造函数里参与了OpenGL设置的初始化。现在CGLView类的对象是CCadSurfView类的私有成员,在 OnCreate ()里对CGLView类对象初 始化, 并在OnDestroy()里删除该对象。通过CGLView的对象调用方法代替了以基类形式直接调用基类的方法操作模式-如下。
CCadSurfView::OnCreate(...)
{
myView = new CGLView(this, GetDocument()->DisplayContext());
}
void CCadSurfView::OnMouseMove(...)
{
.....
myView->RotateView(....);
}
void CCadSurfView::OnSize(...)
{
...
myView->Resize(cx, cy);
CCadSurfView::OnSize.....
...
}
...CCadSurfView::OnDestroy(...)
{
...
delete myView;
...
}
Whereas when it was derived earlier from CView, the calls were like this:而当它早期派生自CView时,调用如下这样:
void CCadSurfView::OnMouseMove(...)
{
...
CGLView::RotateView(...);
...
}