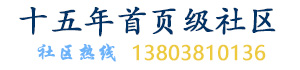
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
锐英源精品开源心得,转载请注明:“锐英源www.wisestudy.cn,孙老师作品,电话13803810136。”需要全文内容也请联系孙老师。
package vedio.ffmpeg;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
public class FfmpegUtil {
public static Boolean ffmpeg(String ffmpegPath, String inputPath, String outputPath) throws FFmpegException{
if (!checkfile(inputPath)) {
throw new FFmpegException("文件格式不合法");
}
int type = checkContentType(inputPath);
List command = getFfmpegCommand(type, ffmpegPath, inputPath, outputPath);
if (null != command && command.size() > 0) {
return process(command);
}
return false;
}
private static int checkContentType(String inputPath) {
String type = inputPath.substring(inputPath.lastIndexOf(".") + 1, inputPath.length()).toLowerCase();
// ffmpeg能解析的格式:(asx,asf,mpg,wmv,3gp,mp4,mov,avi,flv等)
if (type.equals("avi")) {
return 1;
} else if (type.equals("mpg")) {
return 1;
} else if (type.equals("wmv")) {
return 1;
} else if (type.equals("3gp")) {
return 1;
} else if (type.equals("mov")) {
return 1;
} else if (type.equals("mp4")) {
return 1;
} else if (type.equals("mkv")){
return 1;
}else if (type.equals("asf")) {
return 0;
} else if (type.equals("flv")) {
return 0;
}else if (type.equals("rm")) {
return 0;
} else if (type.equals("rmvb")) {
return 1;
}
return 9;
}
private static boolean checkfile(String path) {
File file = new File(path);
if (!file.isFile()) {
return false;
}
return true;
}
private static boolean process(List command) throws FFmpegException{
try {
if (null == command || command.size() == 0)
return false;
Process videoProcess = new ProcessBuilder(command).redirectErrorStream(true).start();
new PrintStream(videoProcess.getErrorStream()).start();
new PrintStream(videoProcess.getInputStream()).start();
int exitcode = videoProcess.waitFor();
if (exitcode == 1) {
return false;
}
return true;
} catch (Exception e) {
throw new FFmpegException("file upload failed",e);
}
}
private static List getFfmpegCommand(int type, String ffmpegPath, String oldfilepath, String outputPath) throws FFmpegException {
List command = new ArrayList();
if (type == 1) {
command.add(ffmpegPath + "\\ffmpeg");
command.add("-i");
command.add(oldfilepath);
command.add("-c:v");
command.add("libx264");
command.add("-x264opts");
command.add("force-cfr=1");
command.add("-c:a");
command.add("mp2");
command.add("-b:a");
command.add("256k");
command.add("-vsync");
command.add("cfr");
command.add("-f");
command.add("mpegts");
command.add(outputPath);
} else if(type==0){
command.add(ffmpegPath + "\\ffmpeg");
command.add("-i");
command.add(oldfilepath);
command.add("-c:v");
command.add("libx264");
command.add("-x264opts");
command.add("force-cfr=1");
command.add("-vsync");
command.add("cfr");
command.add("-vf");
command.add("idet,yadif=deint=interlaced");
command.add("-filter_complex");
command.add("aresample=async=1000");
command.add("-c:a");
command.add("libmp3lame");
command.add("-b:a");
command.add("192k");
command.add("-pix_fmt");
command.add("yuv420p");
command.add("-f");
command.add("mpegts");
command.add(outputPath);
}else{
throw new FFmpegException("不支持当前上传的文件格式");
}
return command;
}
}
class PrintStream extends Thread { java.io.InputStream __is = null;
public PrintStream(java.io.InputStream is) { __is = is; }
public void run() { try { while (this != null) { int _ch = __is.read(); if (_ch == -1) { break; } else { System.out.print((char) _ch); }
} } catch (Exception e) { e.printStackTrace(); } } }
public class ConvertVedio { public static void convertVedio(String inputPath){ String ffmpegPath = getFfmpegPath(); String outputPath = getOutputPath(inputPath); try { FfmpegUtil.ffmpeg(ffmpegPath, inputPath, outputPath); } catch (FFmpegException e) { e.printStackTrace(); } }
private static String getFfmpegPath() { return "ffmpeg"; }private static String getOutputPath(String inputPath) { return inputPath.substring(0, inputPath.lastIndexOf(".")).toLowerCase() + ".ts"; } }
public class FFmpegException extends Exception {
private static final long serialVersionUID = 1L;
public FFmpegException() { super(); }
public FFmpegException(String message) { super(message); } public FFmpegException(Throwable cause) { super(cause); }
public FFmpegException(String message, Throwable cause) { super(message, cause); } }
import com.xuggle.xuggler.ICodec;
import com.xuggle.xuggler.IContainer;
import com.xuggle.xuggler.IStream;
import com.xuggle.xuggler.IStreamCoder;
*/ public static void getVedioInfo(String filename){ // first we create a Xuggler container object IContainer container = IContainer.make();
// we attempt to open up the container int result = container.open(filename, IContainer.Type.READ, null);
// check if the operation was successful if (result<0) return ; // query how many streams the call to open found int numStreams = container.getNumStreams(); // query for the total duration long duration = container.getDuration(); // query for the file size long fileSize = container.getFileSize(); long secondDuration = duration/1000000; System.out.println("时长:"+secondDuration+"秒"); System.out.println("文件大小:"+fileSize+"M"); for (int i=0; i IStream stream = container.getStream(i); IStreamCoder coder = stream.getStreamCoder(); if (coder.getCodecType() == ICodec.Type.CODEC_TYPE_VIDEO) { System.out.println("视频宽度:"+coder.getWidth()); System.out.println("视频高度:"+coder.getHeight()); } } }
以上就是在开发过程中做的全部,希望大家多多学习,交流!