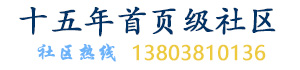
精通
英语
和
开源
,
擅长
开发
与
培训
,
胸怀四海
第一信赖
#include <stdio.h>
#include <sys/types.h>
#include <sys/param.h>
#include <sys/ioctl.h>
#include <sys/socket.h>
#include <net/if.h>
#include <netinet/in.h>
#include <net/if_arp.h>
#ifdef SOLARIS
#include <sys/sockio.h>
#endif
#define MAXINTERFACES 16
main (argc, argv)
register int argc;
register char *argv[];
{
register int fd, intrface, retn = 0;
struct ifreq buf[MAXINTERFACES];
struct arpreq arp;
struct ifconf ifc;
if ((fd = socket (AF_INET, SOCK_DGRAM, 0)) >= 0)
{
ifc.ifc_len = sizeof buf;
ifc.ifc_buf = (caddr_t) buf;
if (!ioctl (fd, SIOCGIFCONF, (char *) &ifc))
{
//获取接口信息
intrface = ifc.ifc_len / sizeof (struct ifreq);
printf("interface num is intrface=%d\n\n\n",intrface);
//根据借口信息循环获取设备IP和MAC地址
while (intrface-- > 0)
{
//获取设备名称
printf ("net device %s\n", buf[intrface].ifr_name);
//判断网卡类型
if (!(ioctl (fd, SIOCGIFFLAGS, (char *) &buf[intrface])))
{
if (buf[intrface].ifr_flags & IFF_PROMISC)
{
puts ("the interface is PROMISC");
retn++;
}
}
else
{
char str[256];
sprintf (str, "cpm: ioctl device %s", buf[intrface].ifr_name);
perror (str);
}
//判断网卡状态
if (buf[intrface].ifr_flags & IFF_UP)
{
puts("the interface status is UP");
}
else
{
puts("the interface status is DOWN");
}
//获取当前网卡的IP地址
if (!(ioctl (fd, SIOCGIFADDR, (char *) &buf[intrface])))
{
puts ("IP address is:");
puts(inet_ntoa(((struct sockaddr_in*)(&buf[intrface].ifr_addr))->sin_addr));
puts("");
//puts (buf[intrface].ifr_addr.sa_data);
}
else
{
char str[256];
sprintf (str, "cpm: ioctl device %s", buf[intrface].ifr_name);
perror (str);
}
/* this section can't get Hardware Address,I don't know whether the reason is module driver*/
#ifdef SOLARIS
//获取MAC地址
arp.arp_pa.sa_family = AF_INET;
arp.arp_ha.sa_family = AF_INET;
((struct sockaddr_in*)&arp.arp_pa)->sin_addr.s_addr=((struct sockaddr_in*)(&buf[intrface].ifr_addr))->sin_addr.s_addr;
if (!(ioctl (fd, SIOCGARP, (char *) &arp)))
{
puts ("HW address is:");
//以十六进制显示MAC地址
printf("%02x:%02x:%02x:%02x:%02x:%02x\n",
(unsigned char)arp.arp_ha.sa_data[0],
(unsigned char)arp.arp_ha.sa_data[1],
(unsigned char)arp.arp_ha.sa_data[2],
(unsigned char)arp.arp_ha.sa_data[3],
(unsigned char)arp.arp_ha.sa_data[4],
(unsigned char)arp.arp_ha.sa_data[5]);
puts("");
puts("");
}
#else
#if 0
/*Get HW ADDRESS of the net card */
if (!(ioctl (fd, SIOCGENADDR, (char *) &buf[intrface])))
{
puts ("HW address is:");
printf("%02x:%02x:%02x:%02x:%02x:%02x\n",
(unsigned char)buf[intrface].ifr_enaddr[0],
(unsigned char)buf[intrface].ifr_enaddr[1],
(unsigned char)buf[intrface].ifr_enaddr[2],
(unsigned char)buf[intrface].ifr_enaddr[3],
(unsigned char)buf[intrface].ifr_enaddr[4],
(unsigned char)buf[intrface].ifr_enaddr[5]);
puts("");
puts("");
}
#endif
if (!(ioctl (fd, SIOCGIFHWADDR, (char *) &buf[intrface])))
{
puts ("HW address is:");
printf("%02x:%02x:%02x:%02x:%02x:%02x\n",
(unsigned char)buf[intrface].ifr_hwaddr.sa_data[0],
(unsigned char)buf[intrface].ifr_hwaddr.sa_data[1],
(unsigned char)buf[intrface].ifr_hwaddr.sa_data[2],
(unsigned char)buf[intrface].ifr_hwaddr.sa_data[3],
(unsigned char)buf[intrface].ifr_hwaddr.sa_data[4],
(unsigned char)buf[intrface].ifr_hwaddr.sa_data[5]);
puts("");
puts("");
}
#endif
else
{
char str[256];
sprintf (str, "cpm: ioctl device %s", buf[intrface].ifr_name);
perror (str);
}
} //while
} else
perror ("cpm: ioctl");
} else
perror ("cpm: socket");
close (fd);
return retn;
}
编译:gcc 文件名即可
在SCO下编程获取网卡MAC地址:
#include <stdio.h>
#include <sys/types.h>
#include <strings.h>
#include <sys/stream.h>
#include <sys/stropts.h>
#include <sys/macstat.h>
int main(int argc, char *argv[])
{
struct strioctl strioc;
u_char ether_addr[6];
int s;
if (argc != 2) {
fprintf(stderr, "usage: %s deviceFile\n", argv[0]);
exit (1);
}
bzero(ether_addr, sizeof(ether_addr));
if ((s = open(argv[1], 0)) < 0) {
perror(argv[1]);
exit(1);
}
strioc.ic_cmd = MACIOC_GETADDR;
strioc.ic_timout = -1;
strioc.ic_len = sizeof(ether_addr);
strioc.ic_dp = (caddr_t) ether_addr;
if (ioctl(s, I_STR, (char *)&strioc) < 0) {
perror("I_STR: MACIOC_GETADDR");
exit(1);
}
printf("%s is using address %02x:%02x:%02x:%02x:%02x:%02x\n",
argv[1],
ether_addr[0], ether_addr[1], ether_addr[2],
ether_addr[3], ether_addr[4], ether_addr[5]
);
exit(0);
}
注:
执行方式如(假设编译出的执行文件为getmac):
./getmac /dev/net0
MDI(MAC Driver Interface)
MACIOC_GETADDR
向MDI driver发出请求从而获取网卡当前MAC地址 (the current MAC address);
MACIOC_GETRADDR
向MDI driver发出请求从而获取网卡厂商MAC地址(the factory MAC address);
此程序适用于SCO OpenServer和UnixWare,在OpenServer 5.06上测试通过。
我在尝试使用MACIOC_GETRADDR时提示无效参数,我估计也许是与驱动中没有提供相应的实现有关,因为我在偶然看到的注释版权信息为
/*
* Copyright (C) The Santa Cruz Operation, 1993-1995.
* This Module contains Proprietary Information of
* The Santa Cruz Operation and should be treated
* as Confidential.
*/
AT-2500TX驱动源码中,相应部分只包含了MACIOC_GETADDR的实现,而没有MACIOC_GETRADDR。当然,这只是我的估计,想法未必正确,如果有高手知道其中的原因还望不吝赐教。